Appearance
Magic Player
Package
@maas/vue-equipment/plugins
Last Changed
4 weeks ago
A magic video player.
Demo
Video Poster [mp4]
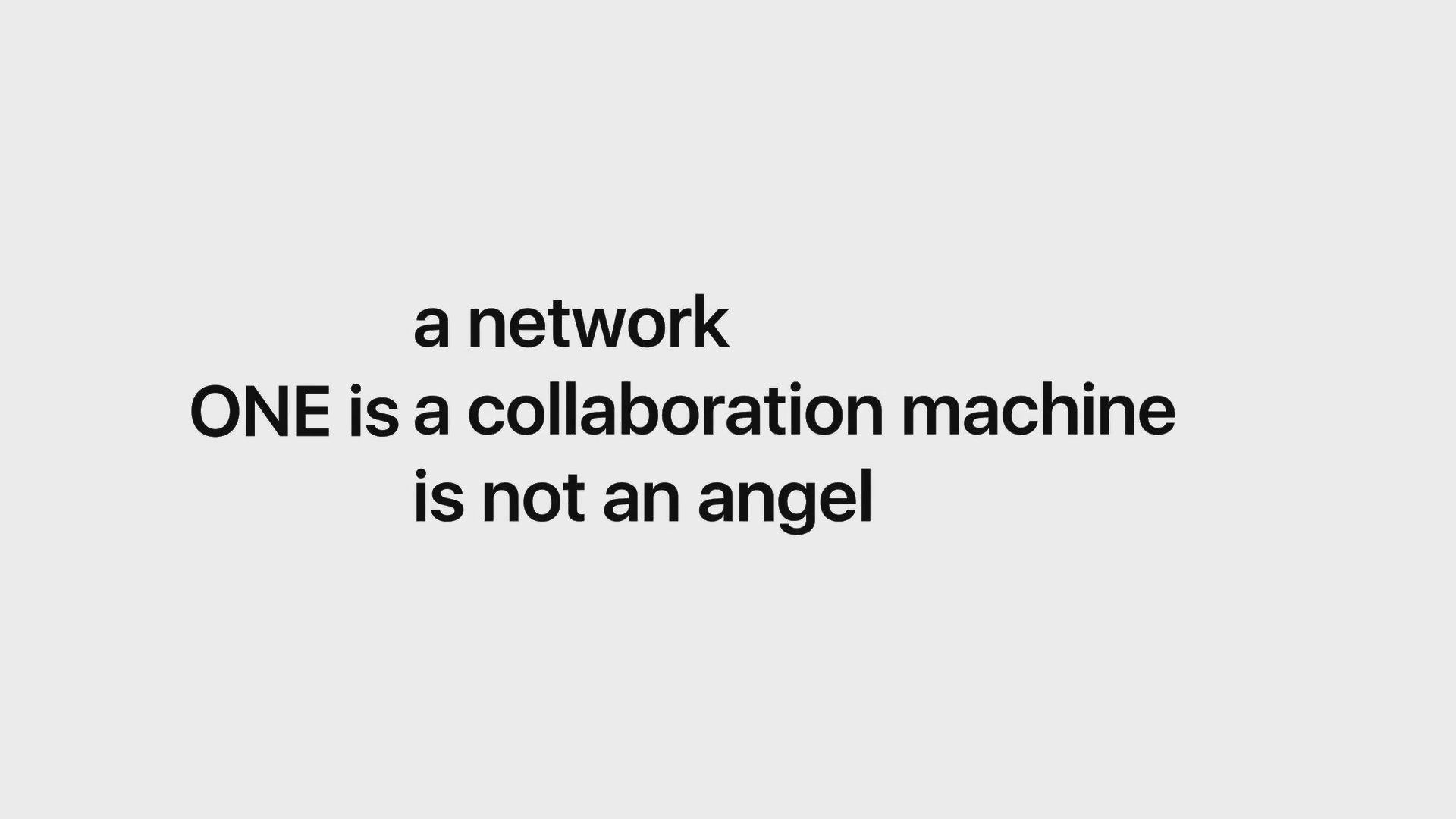
Autoplay [mp4]
Autoplay [hls]
File [mp4]
Popover [mux]
Image Poster [mp4]
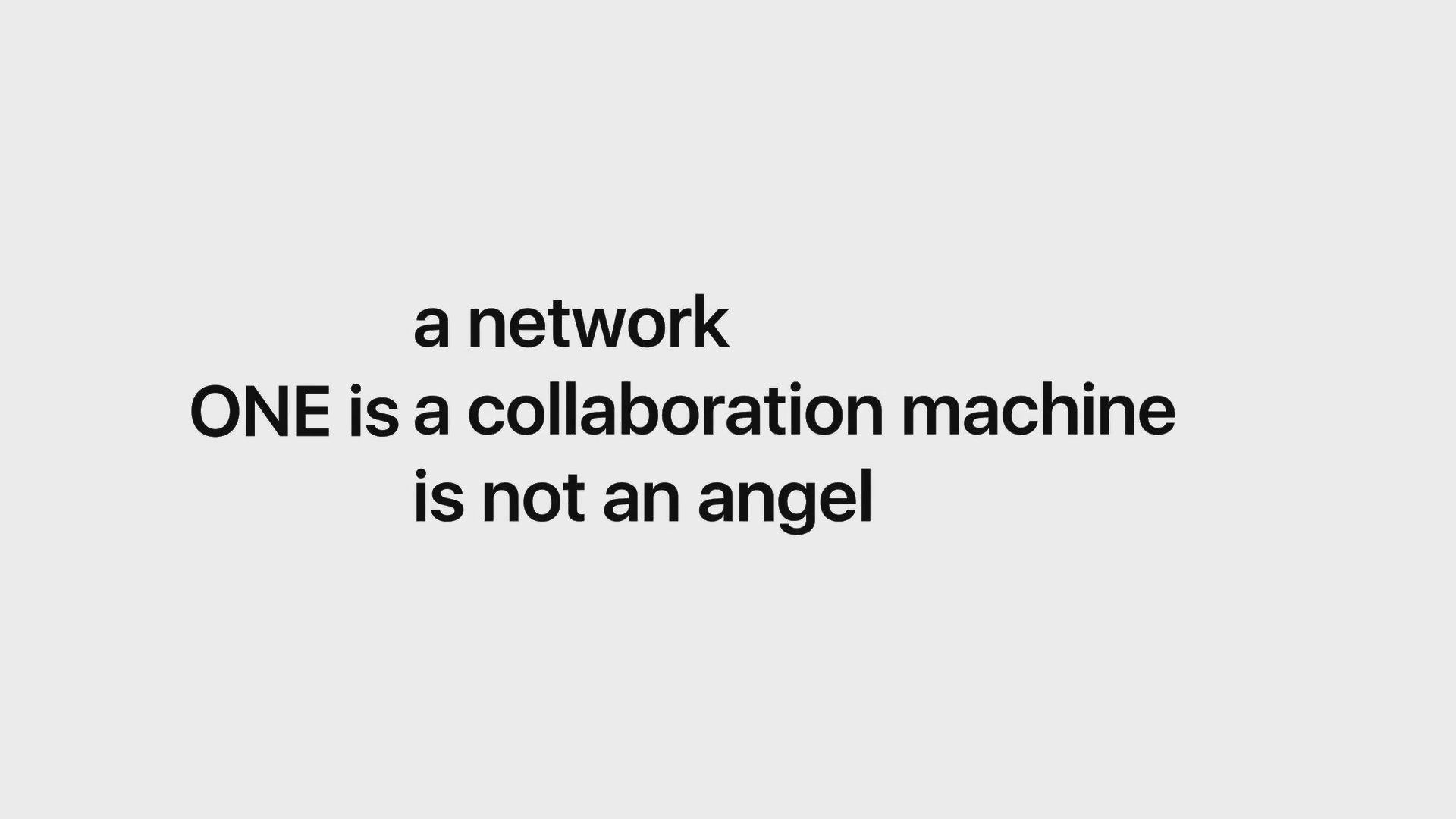
Standalone controls [after]
Standalone controls [before]
Api
Audio Player
Audio Player [slot]
Loveless
Usage
js
import { MagicPlayerPlugin } from '@maas/vue-equipment/plugins'
import { createApp } from 'vue'
const app = createApp({})
app.use(MagicPlayerPlugin)
vue
<template>
<magic-player
src="https://stream.mux.com/c2sidhKoTaKUTgqqACU8AsRRq02uUbEFLrgGQXDjlJks/high.mp4"
>
<magic-player-controls />
</magic-player>
</template>